Interview Questions In Python Programming (2024)
Prepare for your 2024 programming interviews with our curated list of essential Python interview questions, covering basic to advanced concepts and coding challenges.
Python, crafted by the visionary Guido van Rossum and unveiled to the world on February 20, 1991, stands as a beacon of innovation in the programming realm. Celebrated for its extensive application and soaring popularity, Python's interpreted nature seamlessly adapts to dynamic semantics, offering unmatched flexibility.
Embracing the ethos of open-source, Python is a treasure trove of possibilities, free for all to explore. Its elegantly simple and uncluttered syntax is a breath of fresh air, making it an absolute delight for developers to master. Beyond its surface, Python is a powerhouse of object-oriented programming, making it the go-to choice for a myriad of general-purpose programming tasks.
β
The magic of Python lies in its ability to weave complex functionalities into a tapestry of concise code. This efficiency is the cornerstone of Python's meteoric rise in the tech world. Its prowess extends across diverse domains, from the intricate algorithms of Machine Learning and Artificial Intelligence to the creative avenues of Web Development and Web Scraping. Python's robust libraries are the keys to unlocking these powerful computational realms.
β
In a world hungry for technological innovation, Python developers are the sought-after architects of the future. The global demand for their skills is skyrocketing, with companies rolling out the red carpet, adorned with enticing perks and benefits.
β
Embark on a journey with us as we delve into the top Questions and Answers in Python Programming for 2024. This treasure trove of knowledge is your gateway to excelling in your career and seizing those extraordinary job offers.
β
Considering Python's vast applicability, for those looking to hire or be hired in roles requiring Python expertise, Weekday.works offers a streamlined approach to connect with top engineering talent.
Python Programming Interview Questions for Beginners
β
1. What is Python? What are the benefits of using Python?
Python is a high-level, interpreted programming language known for its simplicity, readability, and versatility. It supports multiple programming paradigms, including procedural, object-oriented, and functional programming. Some benefits of using Python include:
- Ease of Learning: Python's syntax is clear and intuitive, making it an excellent choice for beginners.
- Versatility: It can be used for a wide range of applications, from web development to data analysis and machine learning.
- Rich Ecosystem: Python has a vast collection of libraries and frameworks that simplify complex tasks.
- Portability: Python code can run on various platforms without modification.
- Community Support: A large and active community contributes to Python's continuous improvement and offers extensive resources for learning and troubleshooting.
β
2. What is a dynamically typed language?
A dynamically typed language is a programming language where variable types are determined at runtime rather than at compile time. In Python, for example, you don't need to declare the type of a variable explicitly when you assign a value to it; the interpreter infers the type based on the value assigned.
β
3. What is an Interpreted language?
An interpreted language is a type of programming language for which most of its implementations execute instructions directly, without previously compiling a program into machine-language instructions. Python is an example of an interpreted language, where the code is executed line by line, making debugging easier.
β
4. What is Scope in Python?
Scope in Python refers to the region of the code where a variable is accessible. Python has four types of scopes: local, enclosing, global, and built-in, which together form the LEGB rule. Variables defined in different scopes have different accessibility and lifetime within a program.
β
5. What are the common built-in data types in Python?
The common built-in data types in Python include:
- Numeric Types: int, float, complex
- Sequence Types: list, tuple, range
- Mapping Type: dict
- Set Types: set, frozenset
- Boolean Type: bool
- Text Type: str
- Binary Types: bytes, bytearray, memoryview
β
6. What are the differences between expressions and statements
- Expressions: An expression is a combination of values, variables, and operators that yields a result value. For example, 2 + 3 is an expression that evaluates to 5.
- Statements: A statement is an instruction that performs some action but does not necessarily produce a value. For example, print("Hello, World!") is a statement that displays a message.
β
7. What is positive and negative indices in Python
In Python, lists and other sequences can be indexed using both positive and negative numbers. Positive indices start from the beginning of the sequence (0, 1, 2, ...), while negative indices start from the end of the sequence (-1 for the last element, -2 for the second-last element, and so on).
β
8. Exploring the life cycle of a thread in Python
The life cycle of a thread in Python typically includes the following stages:
- Creation: A new thread is created.
- Start: The thread is started and begins executing.
- Running: The thread is actively running its code.
- Waiting: The thread may enter a waiting state if it needs to wait for resources or other conditions.
- Termination: The thread completes its execution and terminates.
β
9. Importance and purpose of PEP 8 in Python
PEP 8 is the official style guide for Python code. It provides guidelines for formatting Python code in a consistent and readable manner, promoting best practices and improving code maintainability.
β
10. What are modules and packages in Python?
- Modules: A module in Python is a file containing Python code, typically defining functions, classes, or variables. Modules allow for code organization and reusability.
- Packages: A package is a collection of Python modules organized in a directory hierarchy. Packages allow for structuring larger code projects and can include subpackages and modules.
β
11. What are global, protected, and private attributes in Python?
- Global attributes: These are variables or functions defined at the top level of a module and are accessible throughout the module and by other modules that import it.
- Protected attributes: By convention, attributes prefixed with a single underscore (_) are considered protected and should not be accessed directly outside the class or module.
- Private attributes: Attributes prefixed with double underscores (__) are considered private and are name-mangled to prevent direct access from outside the class.
β
Python Programming Interview Questions for Intermediates
12. What are the methods for removing spaces from a string
To remove spaces from a string in Python, you can use the following methods:
- Using replace() method: new_string = original_string.replace(" ", "")
- Using join() and split() methods: new_string = "".join(original_string.split())
- Using regular expressions: import re; new_string = re.sub(r"\s+", "", original_string)
β
13. How to display text file contents in reverse order
To display the contents of a text file in reverse order, you can use the following code:
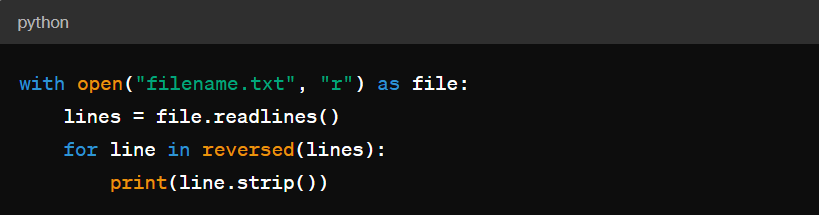
β
14. Python as an object-oriented or functional programming language
Python is primarily an object-oriented programming language, as it supports the creation and manipulation of objects, classes, and inheritance. However, it also supports functional programming concepts such as first-class functions, lambdas, and higher-order functions, making it a versatile language that can be used in a functional programming style.
β
15. Introduce the Flask in Python and its dependencies
Flask is a lightweight web framework for Python that allows you to build web applications quickly and easily. It is designed to be simple and flexible, making it a popular choice for small to medium-sized web projects. Flask's core dependencies include Werkzeug (a WSGI utility library) and Jinja2 (a template engine).
β
16. Understanding Python's tuple-matching feature
Python's tuple-matching feature, also known as tuple unpacking, allows you to assign values from a tuple to multiple variables in a single statement.
β
For example:

This assigns the value 1 to a, 2 to b, and 3 to c.
β
17. What is a break, continue, and pass in Python?
- break: Exits the current loop and resumes execution at the next statement outside the loop.
- continue: Skips the rest of the code inside the loop for the current iteration and moves to the next iteration.
- pass: A no-operation statement that serves as a placeholder where syntactically some code is required, but no action is needed.
β
18. What is the difference between Python Arrays and lists?
- Arrays: In Python, arrays are a collection of elements of the same type. They are provided by the array module and are more efficient for numerical operations.
- Lists: Lists are a more versatile data structure that can hold elements of different types. They are a built-in data type and are used for general-purpose tasks.
β
19. What is slicing in Python?
Slicing in Python is a method to extract a subset of elements from a sequence (such as a list, tuple, or string) by specifying a start, stop, and optional step index.Β
β
For example:

20. What is a docstring in Python?
A docstring is a string literal that occurs as the first statement in a module, function, class, or method definition. It is used to document the purpose and usage of the code. Docstrings are accessible through the __doc__ attribute and the help() function.
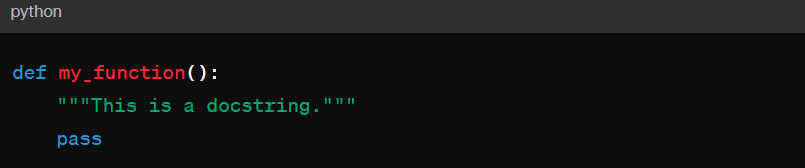
21. What is __init__?
The __init__ method in Python is a special method used for initializing newly created objects. It is called automatically when a new instance of a class is created. It can take arguments to initialize the object's attributes.

Python Programming Interview Questions for Experienced Developers
Preparing for an interview in Python programming? Weekday.works not only helps candidates land their dream job but also offers resources to improve their Python skills. With a community of skilled software engineers, including Python experts, candidates can get practical advice and tips to ace their interviews
22. Compare Pickling and Unpickling Processes
- Pickling: The process of converting a Python object into a byte stream, typically for saving to a file or transmitting over a network. It's done using the pickle.dump() function.
- Unpickling: The reverse process of converting a byte stream back into a Python object. It's done using the pickle.load() function.
23. Differences Between Deep Copy and Shallow Copy
- Shallow Copy: Creates a new object but does not create copies of nested objects. Instead, it just copies the references to the nested objects. Use copy.copy() for this.
- Deep Copy: Creates a new object and recursively copies all nested objects. Use copy.deepcopy() for this.
24. Exploring Python's Memory Management System
Python's memory management system includes:
- Reference Counting: Keeps track of the number of references to each object. When the reference count drops to zero, the object is deallocated.
- Garbage Collection: Identifies and collects cycles of references that are not reachable, preventing memory leaks.
25. What Are Python Namespaces? Why Are They Used?
Namespaces in Python are mappings from names to objects. They are used to avoid naming conflicts and to organize code into logical groups. Examples include local, global, and built-in namespaces.
26. What Are Decorators in Python?
Decorators are a way to modify or enhance functions and methods without changing their code. They are typically used to add functionality, such as logging, timing, or access control.
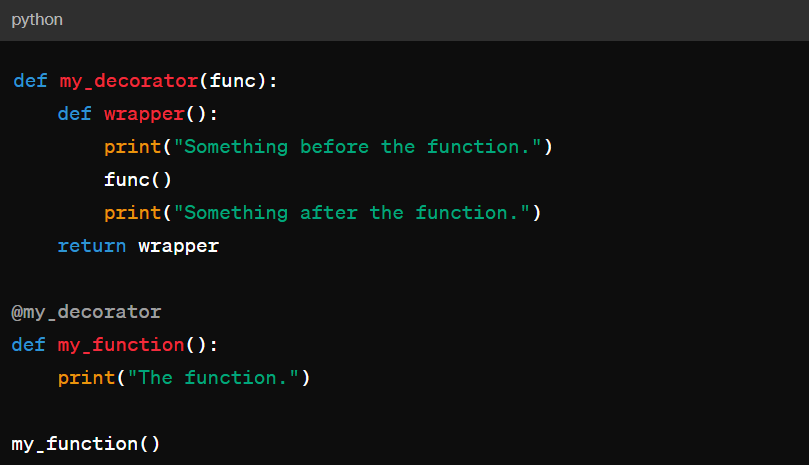
β
27. What Are Dict and List Comprehensions?
Dict and List comprehensions are concise ways to create dictionaries and lists from iterables.
- List Comprehension: [expression for item in iterable]
- Dict Comprehension: {key_expression: value_expression for item in iterable}
β
28. What Is Lambda in Python? Why Is It Used?
Lambda is an anonymous function in Python, defined using the lambda keyword. It is used for creating small, one-time-use functions, often passed as arguments to higher-order functions.
β

β
29. What Is PYTHONPATH in Python?
PYTHONPATH is an environment variable that specifies a list of directories where Python should look for modules when importing them. It's used to add custom or third-party module locations to the import search path.
β
30. How Do You Copy an Object in Python?
You can copy an object in Python using the copy module:
- Shallow Copy: copy.copy(object)
- Deep Copy: copy.deepcopy(object)
β
What Are Generators in Python?
Generators are a type of iterable that generate values on the fly, using the yield keyword. They are memory-efficient and often used for large datasets.

β
Python OOP (Object-Oriented Programming) Interview Questions
β
31. Explane Polymorphism with Examples
Polymorphism is a concept in object-oriented programming that allows objects of different classes to be treated as objects of a common superclass. It enables the same method or property name to be used in different contexts. In Python, polymorphism is often achieved through method overriding or duck typing.
β
Example:
β
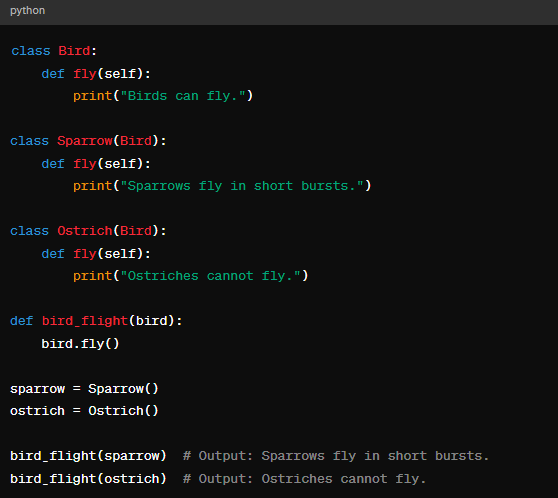
β
32. Characteristics of Python as an Object-Oriented Language
Python is an object-oriented language with the following characteristics:
- Classes and Objects: Python supports the creation of classes and objects for encapsulating data and behavior.
- Inheritance: Python allows classes to inherit attributes and methods from other classes.
- Polymorphism: Python supports polymorphism, allowing different classes to implement the same method in their own way.
- Encapsulation: Python provides mechanisms for restricting access to methods and variables, promoting data hiding.
- Abstraction: Python allows the creation of abstract classes and methods, providing a blueprint for other classes.
β
33. What is Inheritance in Python and Its Types
Inheritance in Python allows a class to inherit attributes and methods from another class. The class that inherits is called the subclass, and the class being inherited from is called the superclass. There are different types of inheritance in Python:
- Single Inheritance: A subclass inherits from one superclass.
- Multiple Inheritance: A subclass inherits from multiple superclasses.
- Multilevel Inheritance: A subclass inherits from a superclass, which in turn inherits from another superclass.
- Hierarchical Inheritance: Multiple subclasses inherit from a single superclass.
Example:
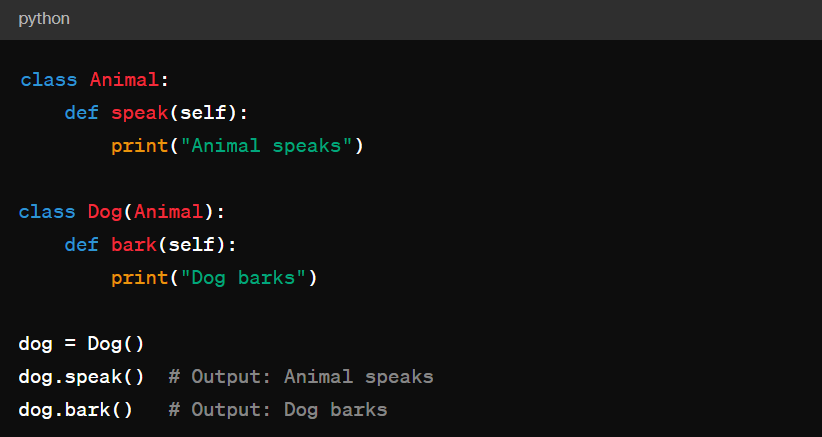
β
34. Implement Access Specifiers in Python
Access specifiers in Python determine the accessibility of class members. Python has three types of access specifiers:
- Public: Accessible from anywhere.
- Protected: Accessible within the class and its subclasses. Indicated by a single underscore (_).
- Private: Accessible only within the class. Indicated by a double underscore (__).
β
Example:
β
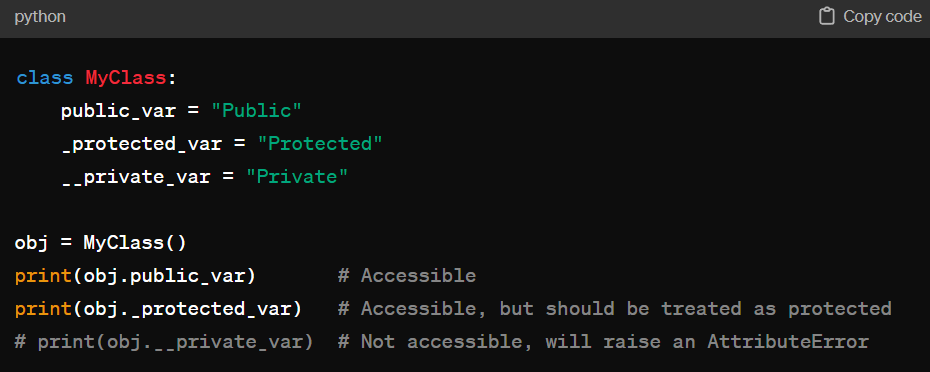
β
35. How to Create an Empty Class Using the Pass Keyword
In Python, the pass keyword is used to create an empty class, which can later be filled with attributes and methods.
Example:
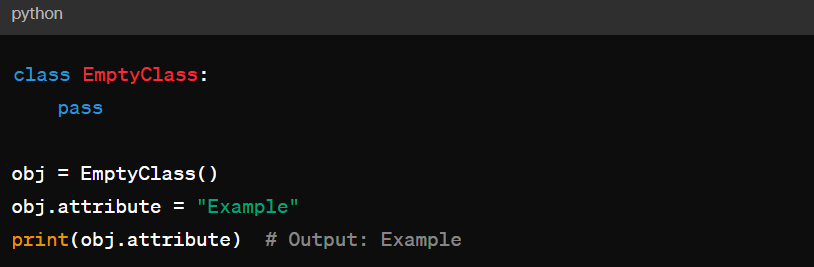
β
36. How Does Inheritance Work in Python? Explain It With an Example.
Inheritance in Python allows a subclass to inherit attributes and methods from a superclass. The subclass can also override or extend the functionality of the superclass.
β
Example:
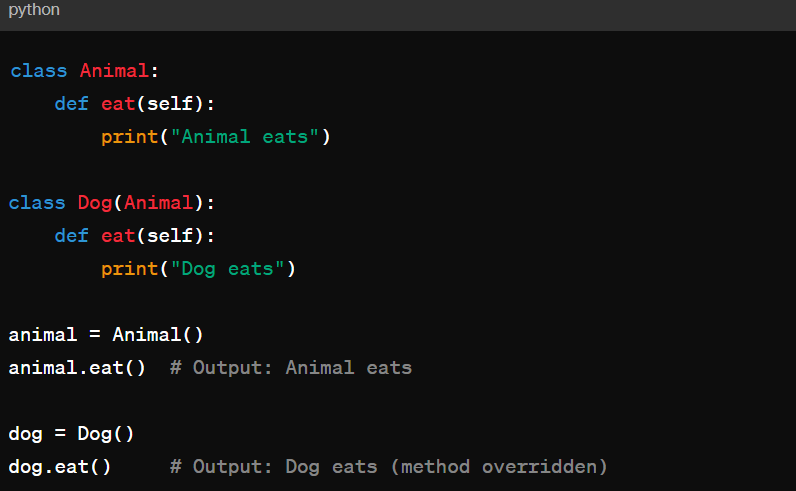
β
37. Is It Possible to Call a Parent Class Without Its Instance Creation?Β
Yes, it is possible to call a parent class without creating its instance by using the super() function in the subclass.
β
38. Differentiate Between New and Override Modifiers.
- new modifier: Used to create a new instance of a class.
- override modifier: Used to provide a new implementation for an inherited method.
β
Example:

β
Advanced Python Scripting Interview Questions
Practical scripting questions for enhancing Python shell scripting skills
β
1. How can you use Python to automate repetitive system administration tasks?
Python's os and subprocess modules can be used to interact with the operating system, execute shell commands, and automate tasks like file management, process management, and system monitoring. Scripts can be written to automate backups, monitor system health, and perform other routine tasks.
β
2. Write a Python script to monitor a specific directory for any file changes and send an email notification if a change is detected.
The watchdog library can be used to monitor directory changes, and the smtplib library can be used to send email notifications. The script would involve setting up a watchdog observer to watch for file events and then sending an email using smtplib when changes are detected.
β
3. How would you implement a simple web server in Python for serving static files?
Python's built-in http.server module can be used to create a basic HTTP server. You can use the SimpleHTTPRequestHandler class to serve static files from a directory. This can be useful for quick testing or prototyping.
β
4. Write a Python script to parse a log file and extract error messages with their corresponding timestamps.
Regular expressions with the re-module can be used to parse log files and extract specific patterns, such as error messages and timestamps. The script would involve reading the log file line by line, applying the regex pattern, and extracting the required information.
β
5. How can you use Python to perform network automation tasks, such as configuring network devices or checking network connectivity?
Libraries like netmiko can be used for SSH connections to network devices, paramiko for general SSH connectivity, and ping for checking network connectivity. Python scripts can automate tasks such as configuring network devices, checking device status, and running diagnostic tests.
β
6. Write a Python script to compress and decompress files using the gzip or zipfile modules.
The gzip or zipfile modules can be used to compress and decompress files. The script would involve using these modules to create compressed archives of files or directories and to extract files from these archives.
β
7. How would you use Python to interact with APIs for data retrieval and automation tasks?
The requests library can be used to make HTTP requests to REST APIs, parse JSON responses, and automate tasks such as data retrieval or triggering actions on remote services. Python scripts can be written to interact with various APIs, including web services, cloud platforms, and custom APIs.
β
8. Write a Python script to automate database backups and restoration processes.
Python's database libraries (e.g., sqlite3, psycopg2 for PostgreSQL, pymysql for MySQL) can be used to connect to databases, execute backup commands, and restore data from backup files. The script would involve connecting to the database, running backup commands, and storing the backup files in a secure location.
β
9. How can you use Python to automate testing of web applications?
The selenium library can be used to automate web browser interactions for testing purposes, such as filling forms, clicking buttons, and verifying page content. Python scripts can be written to automate various test scenarios and validate the functionality of web applications.
β
10. Write a Python script to monitor system resources (CPU, memory, disk usage) and alert if certain thresholds are exceeded.
The psutil library can be used to gather system resource information. The script would involve periodically checking the CPU, memory, and disk usage, and sending alerts (e.g., email, log messages) when specified thresholds are exceeded.
β
Conclusion
In 2024, proficiency in Python remains crucial for tech-related roles. Preparing for interviews involves mastering core concepts and staying updated with language advancements. Practice a variety of questions, understand Python's practical applications, and showcase your skills through projects or certifications. Continuous learning and adaptation are essential for success in the ever-evolving tech landscape.
β
FAQsΒ
β
Looking to join organizations like Google, Spotify, or Netflix? Weekday.works connects you with top companies seeking Python expertise. By becoming part of Weekday.works, you gain access to a network that could open doors to career opportunities in tech giants and startups alike
β
The Importance of Practicing Interview Questions
Practicing interview questions is crucial for several reasons. It helps you familiarize yourself with the types of questions you might encounter, improves your problem-solving skills, and reduces interview anxiety. Regular practice can also help you identify areas where you need improvement and build confidence in your abilities.
Difficulty Levels of Python Programming Interviews
The difficulty levels of Python programming interviews can vary depending on the position and the company. Entry-level positions might focus on basic syntax, data structures, and simple algorithms. Mid-level positions may require a deeper understanding of algorithms, data structures, and system design. Senior positions often involve complex problem-solving, design patterns, and architecture questions.
Organizations Commonly Using Python
Python is used by a wide range of organizations, from startups to tech giants. Some well-known companies that use Python include Google, Facebook, Instagram, Spotify, Netflix, and Dropbox. It's also used in various industries such as finance, healthcare, and academia for data analysis, web development, machine learning, and more.
Reasons Behind Python's Widespread Popularity
Python's popularity can be attributed to several factors:
- Simplicity and Readability: Python's syntax is clean and readable, making it easy to learn and use.
- Versatility: Python can be used for web development, data analysis, machine learning, automation, and more.
- Strong Community: Python has a large and active community, providing extensive resources and libraries.
- Compatibility: Python is platform-independent and can run on various operating systems.
Ways to Improve Python Skills Through Courses or Certifications
Improving your Python skills can be achieved through various means:
- Online Courses: Platforms like Coursera, Udemy, and edX offer Python courses for all skill levels.
- Certifications: Obtaining certifications like the Python Institute's PCEP or PCAP can validate your skills.
- Practice Platforms: Websites like LeetCode, HackerRank, and Codecademy provide practice exercises and challenges.
- Personal Projects: Building your own projects can help you apply your skills and learn new concepts.